Complete Python Scripting and Automation Tutorial for Beginners
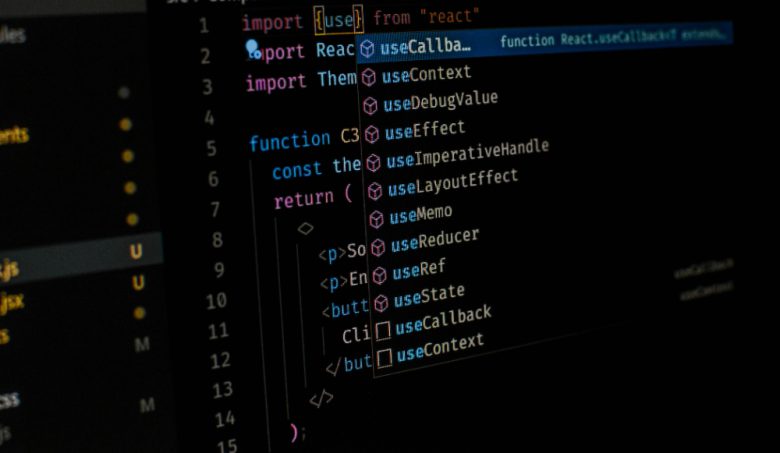
Python has become one of the most popular programming languages for scripting and automation due to its simplicity and versatility. Whether you’re a beginner or an experienced developer, Python offers powerful tools for automating repetitive tasks that save time and increase productivity. From file processing and web scraping to managing databases and sending emails, Python scripts are a must-have in today’s tech-driven world.
This tutorial will guide you through the basics of Python scripting and automation and help you take the first steps towards mastering these important techniques.
What is Python Scripting and Automation?
Python scripting refers to writing small programs, called scripts, to perform specific tasks. These scripts are designed to automate repetitive or time-consuming processes, making workflows faster and more efficient.
Automation with Python involves using its libraries and tools to handle tasks such as file management, data analysis, web scraping, and email automation. By writing Python scripts, you can reduce manual work and focus on more important projects, making it a valuable skill for beginners and professionals alike.
Getting Started
To begin with Python scripting and automation, you first need to install Python on your system. Visit python.org and download the latest version for your operating system. Once installed, you can write and run your scripts using a code editor like VS Code or PyCharm.
Start with a simple script to test your setup:
print("Hello, World!")
Important Python Libraries for Automation
Python provides a vast collection of libraries that make automating tasks simple and powerful. These libraries handle everything from file management and web interactions to data analysis and task scheduling. Below are some of the most important Python libraries for automation:
1. os and shutil
These built-in libraries are essential for managing files and directories.
import os
import shutil
# Create a new folder
os.mkdir("example_folder")
# Copy a file into the newly created folder
shutil.copy("source.txt", "example_folder/target.txt")
2. Selenium
Selenium is a powerful tool for automating web browsers. It allows you to simulate user interactions like clicking buttons, filling out forms, or navigating websites.
Example Use Cases:
Automate login processes.
Test web applications.
Scrape dynamic content.
from selenium import webdriver
# Initialize a browser driver (make sure ChromeDriver is installed)
driver = webdriver.Chrome()
# Open a webpage
driver.get("https://example.com")
# Find an element by its ID and simulate a click
driver.find_element_by_id("login").click()
# Close the browser
driver.quit()
3. requests and BeautifulSoup
These libraries are essential for web scraping and extracting data from websites.
requests: Fetches HTML content from web pages.
BeautifulSoup: Parses HTML to extract specific elements, such as headings, links, or tables.
import requests
from bs4 import BeautifulSoup
# Fetch the HTML content of the webpage
response = requests.get("https://example.com")
# Parse the HTML using BeautifulSoup
soup = BeautifulSoup(response.text, "html.parser")
# Print the title of the webpage
print(soup.title.text)
import pandas as pd
# Read data from an Excel file
data = pd.read_excel("data.xlsx")
# Add a new column with manipulated values
data["new_column"] = data["existing_column"] * 2
# Save the updated data back to an Excel file
data.to_excel("updated_data.xlsx", index=False)
import schedule
import time
# Define a task to be executed
def job():
print("Task executed!")
# Schedule the task to run every 10 seconds
schedule.every(10).seconds.do(job)
# Keep the script running to check for scheduled tasks
while True:
schedule.run_pending()
time.sleep(1)
6. pyautogui
pyautogui is a Python library for automating graphical user interfaces (GUIs). It lets you control the mouse and keyboard programmatically, making it ideal for tasks like filling forms, clicking buttons, or automating repetitive actions in desktop applications.
Key Features
Mouse Control: Move, click, and drag the mouse.
Keyboard Control: Type text, press keys, or simulate key combinations (e.g.,
Ctrl+C
).Screen Interaction: Take screenshots and locate images on the screen.
import pyautogui
# Types with a slight delay
pyautogui.write("Hello, World!", interval=0.1)
# Simulates pressing 'Enter'
pyautogui.press("enter")
# Moves the mouse to (500, 500) in 1 second
pyautogui.moveTo(500, 500, duration=1)
# Left-clicks
pyautogui.click()
Best Practices and Tips
To get the most out of Python scripting and automation, it’s essential to follow best practices and utilize helpful tips. These can improve efficiency, maintainability, and reliability in your automation scripts.
1. Start with Simple Scripts
Begin with small, manageable scripts to automate repetitive tasks. Gradually add complexity as you become comfortable with Python and automation libraries.
2. Use Virtual Environments
Create virtual environments to isolate dependencies for each project. This avoids conflicts between different libraries or versions. Example:
python -m venv myenv
source myenv/bin/activate
3. Leverage Built-in Libraries
Take advantage of Python’s extensive standard library for file management (os
, shutil
), task scheduling (time
, schedule
), and other tasks before exploring third-party tools.
4. Write Modular Code
Break your script into functions or modules. This makes your code reusable and easier to debug or update.
def read_file(file_path):
with open(file_path, 'r') as file:
return file.read()
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
5. Handle Errors Gracefully
Use try-except blocks to handle errors and keep your automation scripts running without crashing. Example:
try:
result = 10 / 0
except ZeroDivisionError:
print("Error: Division by zero!")
6. Log Your Script’s Actions
Implement logging to track your script’s behavior and debug issues efficiently. Example:
import logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(message)s')
logging.info("Script started")
7. Test Scripts Before Automating Critical Tasks
Always test your scripts in a controlled environment before running them on critical or sensitive data.
8. Document Your Scripts
Add comments and docstrings to explain what your script does, making it easier for others (or future you) to understand.
9. Optimize and Refactor Regularly
Review and improve your scripts periodically to ensure they remain efficient and maintainable.
Additional Learning Resources
To dive deeper into Python scripting and automation, you can leverage high-quality resources that provide step-by-step tutorials and real-world projects. One of the best resources for beginners is the book Automate the Boring Stuff with Python.
Why This Book?
Beginner-Friendly: Designed for those new to Python, offering clear explanations and hands-on projects.
Real-World Use Cases: Learn how to automate tasks like data entry, web scraping, and file management.
Practical Examples: Covers useful libraries like
pyautogui
,Selenium
, andPandas
.
Topics Covered in the Book
Automating file and folder management.
Web scraping and browser automation.
Automating Excel and PDF tasks.
Writing scripts for repetitive tasks like email handling.
Get Your Copy
Start automating today with: 👉 Automate the Boring Stuff with Python – Click Here
Conclusion
Python scripting and automation are essential skills for streamlining repetitive tasks and boosting productivity. By mastering libraries like os
, requests
, Selenium
, and pyautogui
, you can automate everything from file management to web scraping. Start with simple scripts, follow best practices, and continuously improve your skills. With resources like Automate the Boring Stuff with Python, you can quickly become proficient and save time in your daily tasks. Start automating today and unlock the power of Python!