Master Python WebSocket Programming for Real-Time Communication: A Complete Beginner’s Guide
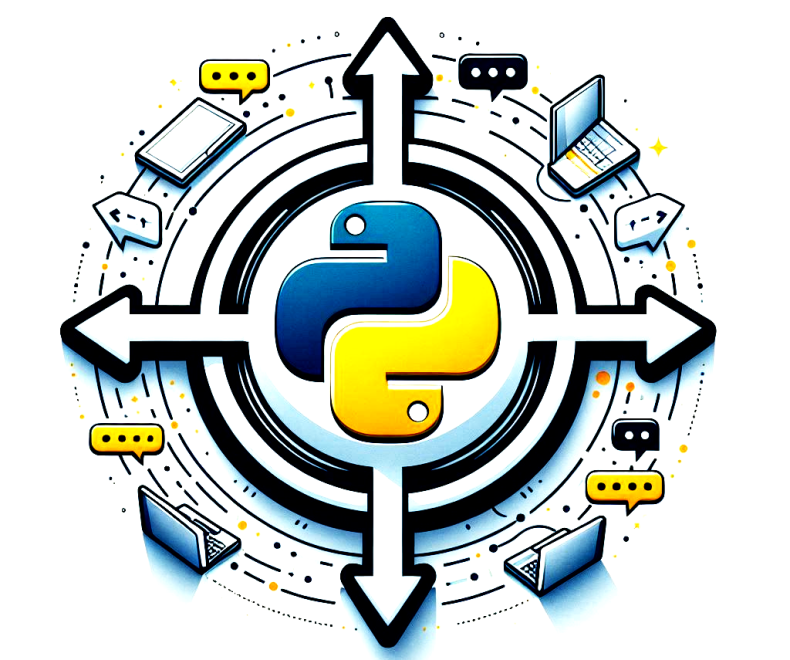
WebSockets enable real-time, two-way communication between clients and servers, making them essential for applications like live chats, notifications, and multiplayer games. Unlike traditional HTTP, WebSockets maintain a continuous connection, delivering faster and more interactive experiences.
Python, with its robust libraries, makes WebSocket programming simple and powerful. In this guide, we’ll explore how to use Python to create real-time applications, covering setup, core concepts, and a step-by-step example. By the end, you’ll be ready to build dynamic, real-time systems with ease.
What Are WebSockets?
WebSockets are a standardized communication protocol (RFC 6455) that allows persistent, bidirectional connections between a client and a server. Unlike traditional HTTP, where requests and responses are separate, WebSockets maintain a continuous connection, enabling real-time data exchange without repeated handshakes.
This protocol is ideal for applications requiring instant updates, such as chat systems, live notifications, stock market tickers, and multiplayer games. WebSockets support subprotocols like STOMP or AMQP for structured messaging and can operate over secure connections (WSS) to ensure data privacy and integrity.
By reducing latency and overhead, WebSockets outperform polling or long-polling methods. Their efficiency and compatibility with modern web browsers and servers make them a cornerstone of real-time communication at scale.
Python makes WebSocket programming simple with libraries like websockets
and Socket.IO
, which streamline setup and integration. These tools enable efficient, real-time communication for interactive web applications.
Setting Up Your Environment
Before diving into Python WebSocket programming, you need to set up your environment. First, ensure you have Python installed (version 3.6 or later). Next, you’ll need to install a WebSocket library, such as websockets
, which simplifies handling WebSocket connections. You can install it using pip:
pip install websockets
This library provides an easy-to-use interface for building WebSocket servers and clients, making it ideal for creating real-time applications in Python. Once you’ve installed the necessary library, you’re ready to start coding your WebSocket application.
Building a Real-Time Chat Application
Websocket Server in Python
To set up a basic WebSocket server in Python, we use the websockets
library to handle connections. The server is designed to maintain persistent, two-way communication between the client and server, which makes it ideal for real-time applications like chat systems.
In this example, the server listens on localhost
at port 8765
. It uses asyncio
, a library that helps manage asynchronous operations, allowing the server to handle multiple client connections simultaneously without blocking. The connected_clients
set keeps track of all the active WebSocket connections, ensuring that messages can be broadcast to all clients.
When a client connects, the server adds their connection to the connected_clients
set. The chat
function listens for incoming messages from the client with async for message in websocket
, and when a message is received, it sends the message to all other connected clients (except the one that sent it). This is done in real time, so as soon as one client sends a message, all other connected clients will receive it almost instantly. Once a client disconnects, their WebSocket object is removed from the connected_clients
set to ensure no further messages are sent to them.
import asyncio
import websockets
connected_clients = set()
async def chat(websocket, path):
connected_clients.add(websocket)
try:
async for message in websocket:
for client in connected_clients:
if client != websocket:
await client.send(message)
finally:
connected_clients.remove(websocket)
start_server = websockets.serve(chat, "localhost", 8765)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
In this basic setup, the server listens for incoming WebSocket connections on localhost
port 8765
. It keeps track of connected clients and relays messages to all other clients in real time.
Websocket Client Python
Here’s how to build the Python WebSocket client that connects to the server we created earlier:
import asyncio
import websockets
connected_clients = set()
async def chat(websocket, path):
connected_clients.add(websocket)
try:
async for message in websocket:
for client in connected_clients:
if client != websocket:
await client.send(message)
finally:
connected_clients.remove(websocket)
start_server = websockets.serve(chat, "localhost", 8765)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
This client connects to the WebSocket server and allows the user to send and receive messages in real time. The send_message()
function waits for user input and sends it to the server, while the receive_message()
function continuously listens for incoming messages from the server and prints them to the console.
With this setup, you can have multiple clients connected to the server at once. When one client sends a message, it gets broadcast to all other connected clients, allowing for a simple but effective real-time chat experience.
Advanced WebSocket Features
WebSocket offers several advanced features that enhance the functionality and performance of real-time applications. These include:
Ping-Pong Mechanism: This feature ensures the connection is alive by sending periodic ping messages from the server to the client and vice versa. If there’s no response, the connection can be closed, which helps maintain stable communication.
Connection Handling: WebSockets allow you to manage connections effectively by tracking active users and handling scenarios like connection drops or reconnections. You can also set timeouts for idle connections to avoid unnecessary resource usage.
Secure WebSockets (wss://): For secure communication over WebSockets, you can use the
wss://
protocol instead ofws://
. This ensures the data transmitted between client and server is encrypted and protected from unauthorized access.
Conclusion
Python WebSocket Programming for Real-Time Communication is an efficient and effective way to build real-time, interactive applications. With libraries like websockets, setting up a WebSocket server and client is simple, enabling fast, two-way communication for applications like chat systems and live updates. Advanced features such as ping-pong messages, connection handling, and secure WebSockets further enhance the reliability and security of your applications. By mastering Python WebSocket Programming for Real-Time Communication, you can create scalable, real-time systems with ease.