Convolutional Neural Networks - Stock Market Predictions: A Python Tutorial
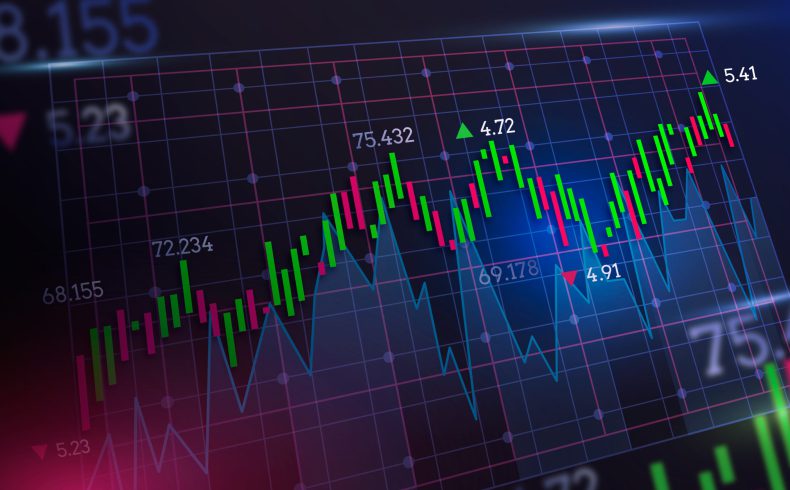
In this article, we will show you how to develop Convolutional Neural Networks (CNNs) for Stock Market Prediction using Python. We will walk you through the steps of analyzing stock price movements with CNNs, leveraging the powerful frameworks Keras and TensorFlow. To train the model, we will use historical stock price data retrieved through the Yahoo Finance API.
Required Python libraries
Make sure you have the following Python libraries installed or download the Github Repository:
pip install numpy pandas matplotlib yfinance tensorflow scikit-learn
NumPy: For numerical calculations.
Pandas: For managing data.
Matplotlib: For visualising the data.
finance: For retrieving historical share price data.
TensorFlow and Keras: For creating and training the CNN.
Scikit-learn: For data preparation and splitting.
Step 1: Fetching and Preparing Stock Market Dat
First, we need to retrieve historical stock price data from a source like Yahoo Finance. Here we use the yfinance library to download the data for a specific stock, e.g., Apple (AAPL).
import yfinance as yf
import pandas as pd
# Fetch stock data
symbol = 'AAPL'
data = yf.download(symbol, start="2015-01-01", end="2024-01-01")
# Use relevant columns
data = data[['Open', 'High', 'Low', 'Close', 'Volume']]
Step 2: Data Preprocessing
For the CNN, we need to transform the time-series data into a suitable format by creating sliding windows of cumulative stock prices.
import numpy as np
from sklearn.preprocessing import MinMaxScaler
# Scale data for better CNN performance
scaler = MinMaxScaler(feature_range=(0, 1))
data_scaled = scaler.fit_transform(data['Close'].values.reshape(-1, 1))
# Create sequences for time windows
def create_dataset(data, time_window):
X, y = [], []
for i in range(len(data) - time_window):
X.append(data[i:i+time_window])
y.append(data[i+time_window])
return np.array(X), np.array(y)
time_window = 60 # 60-day window
X, y = create_dataset(data_scaled, time_window)
# Split into training and testing sets
train_size = int(len(X) * 0.8)
X_train, X_test = X[:train_size], X[train_size:]
y_train, y_test = y[:train_size], y[train_size:]
Step 3: Building the CNN Model
We’ll design a CNN with layers that extract temporal patterns from stock price sequences.
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv1D, MaxPooling1D, Flatten, Dense
# Build the CNN model
model = Sequential()
# Convolutional layer
model.add(Conv1D(filters=64, kernel_size=3, activation='relu', input_shape=(X_train.shape[1], 1)))
model.add(MaxPooling1D(pool_size=2))
# Additional convolutional layer
model.add(Conv1D(filters=32, kernel_size=3, activation='relu'))
model.add(MaxPooling1D(pool_size=2))
# Flatten and dense layers
model.add(Flatten())
model.add(Dense(50, activation='relu'))
model.add(Dense(1)) # Output layer
# Compile the model
model.compile(optimizer='adam', loss='mean_squared_error')
Step 4: Training the CNN
Train the model on the training dataset and validate it on the testing dataset.
# Reshape data for training
X_train = X_train.reshape(X_train.shape[0], X_train.shape[1], 1)
X_test = X_test.reshape(X_test.shape[0], X_test.shape[1], 1)
# Train the model
model.fit(X_train, y_train, epochs=10, batch_size=32, validation_data=(X_test, y_test))
Step 5: Predicting and Visualizing Results
Use the trained CNN to make predictions and compare them with actual prices.
# Make predictions
predictions = model.predict(X_test)
# Rescale predictions back to original scale
predictions = scaler.inverse_transform(predictions)
y_test_rescaled = scaler.inverse_transform(y_test.reshape(-1, 1))
# Visualize the results
import matplotlib.pyplot as plt
plt.plot(y_test_rescaled, color='blue', label='Real Stock Price')
plt.plot(predictions, color='red', label='Predicted Stock Price')
plt.title('Stock Price Prediction using CNN')
plt.xlabel('Time')
plt.ylabel('Stock Price')
plt.legend()
plt.show()
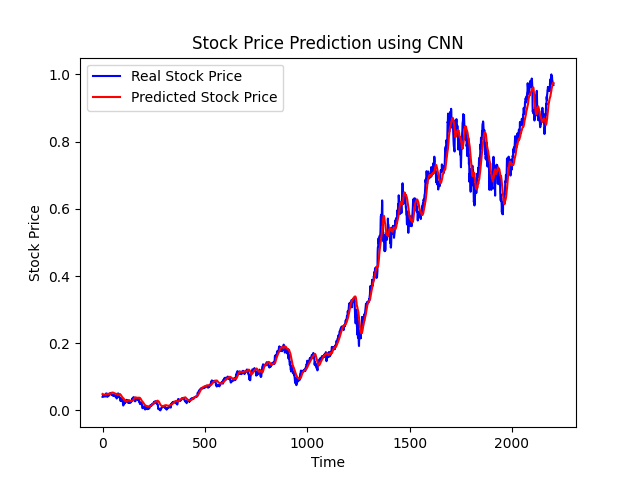
Step 6: Improving the Model
Incorporate Additional Features: Add trading volume, moving averages, or other financial indicators.
Optimize Hyperparameters: Experiment with the number of layers, filters, kernel sizes, and learning rates.
Use External Datasets: Include news sentiment or economic data for enhanced predictions.
Cross-Validation: Apply cross-validation to test the model’s robustness.
Potential Improvements: Enhancing the Stock Prediction Model
1. Incorporate Additional Features
Enhance the dataset by adding indicators like trading volume, moving averages, RSI, or Bollinger Bands. These features provide a more detailed view of market dynamics and can improve prediction accuracy.
2. Optimize Hyperparameters
Experiment with the number of layers, filter sizes, kernel dimensions, and learning rates. Using tools like Keras Tuner or Optuna can streamline the optimization process and identify the best configuration for your model.
3. Use External Datasets
Integrate external data such as news sentiment, macroeconomic factors (e.g., GDP, interest rates), or even social media trends. These additional data sources can capture factors influencing stock prices that aren’t reflected in historical prices alone.
4. Cross-Validation
Implement K-Fold or Walk-Forward validation to test the model’s performance across different time periods. This approach ensures the model’s robustness and its ability to generalize well to unseen data.
Advantages and Disadvantages of Using Convolutional Neural Networks for Stock Market Prediction
Advantages
Captures Temporal Patterns: CNNs excel at recognizing patterns in sequential data, making them effective for analyzing trends and fluctuations in stock prices.
Feature Extraction: The ability of CNNs to automatically extract relevant features from raw data reduces the need for manual feature engineering.
Scalability: CNNs can handle large datasets, which is critical in stock market analysis that involves high-frequency trading or extensive historical data.
Adaptability: The model can be extended with additional features, like news sentiment or other market indicators, for more comprehensive predictions.
Disadvantages
Lack of Causality Understanding: CNNs focus on correlations, not causal relationships, which might lead to misleading predictions if external factors suddenly shift (like political events).
Data Sensitivity: The model’s performance heavily relies on the quality and quantity of input data. Poor or insufficient data can lead to inaccurate results.
Overfitting Risk: Without proper regularization and validation, the model may overfit to training data, failing to generalize well on unseen data.
Complexity and Computation: CNNs require significant computational resources for training and optimization, which might be a barrier for some users.
Conclusions
CNNs are a powerful tool for predicting stock market trends, leveraging patterns in historical data for insightful analysis. With Python and libraries like TensorFlow, creating these models is accessible to both beginners and experts. While they excel at feature extraction and scalability, challenges like overfitting and market volatility remain. By optimizing features and integrating external data, CNNs can provide actionable insights into stock price movements.