Modern API Design Guidelines for 2025: Best Practices with Python Example
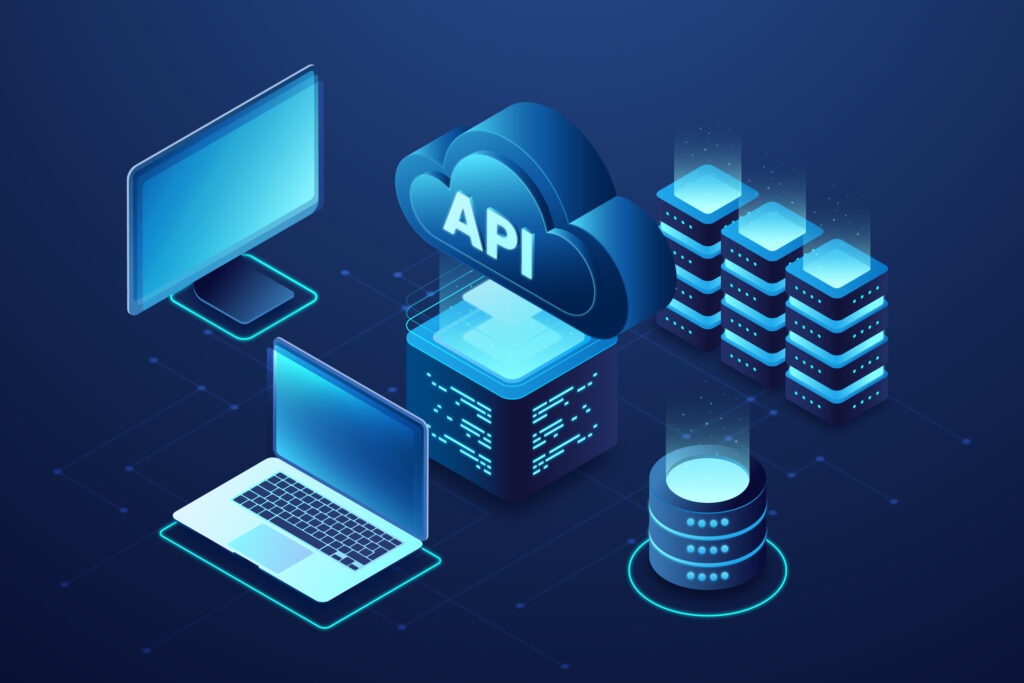
APIs are the unsung heroes of modern software development. They connect applications, enable innovative features, and drive digital transformation. But what truly makes an API exceptional? The answer lies in its design.
In 2025, good API design is more important than ever. Businesses and developers must adapt to growing demands such as scalability, security, and user-friendliness. APIs are no longer just tools—they are products designed to attract and retain developers.
In this article, we’ll explore how to design a modern, well-structured API that meets the demands of the future. We’ll share best practices, provide a Python example, and demonstrate how to document and deploy APIs effectively.
Why API Design Matters
APIs are at the heart of modern software development, playing a critical role in enabling seamless communication between applications and microservices. They allow data exchange and functionality integration across diverse systems—from mobile apps to cloud-based solutions.
Good API design is more than a technical necessity—it’s an enabler of collaboration, a reducer of development efforts, and a guarantee that systems remain stable and flexible under growing demands. Here’s why API design is crucial:
Enhanced Developer Experience
An intuitive, well-documented API empowers developers to implement features faster, reducing development time and costs.Scalability and Performance
A thoughtfully designed API handles high traffic and complex operations efficiently—essential for modern applications and microservices.Interoperability
APIs adhering to standards like REST or GraphQL facilitate seamless integration with external systems, opening doors to new business opportunities.Security
Robust API design incorporates strong authentication and data protection mechanisms, minimizing risks and building user trust.Future-Proofing
A flexible API design simplifies adaptation to emerging technologies and evolving requirements without overhauling the architecture.
By focusing on these aspects, you can create APIs that not only meet today’s challenges but also stand the test of time.
Best Practices for API Design in 2025
Modern APIs need to be flexible, efficient, and easy to integrate. These foundational principles will help you create APIs that meet today’s demanding standards:
1. Choosing the Right API Type
Selecting the appropriate API type depends on the specific use case. While RESTful APIs remain the most widely used, technologies like GraphQL, gRPC, and WebSockets provide distinct advantages in specialized scenarios:
- RESTful APIs: Great for straightforward applications that rely on standardized communication.
- GraphQL: Ideal for complex data queries, especially when the client needs control over the response structure.
- gRPC: A top choice for high-performance systems and microservice communication.
- WebSockets: Perfect for real-time applications like chat systems or live data updates.
2. Consistent and Intuitive Design
An API’s design should be logical and consistent, making it easy for developers to work with:
- Clear URL Structures: Use URLs that clearly reflect resources, such as
/users
for user data or/orders/{id}
for specific orders. - Descriptive Naming: Choose meaningful names to minimize confusion.
3. Prioritizing Developer Experience
A great API isn’t just functional—it’s also enjoyable to use. Developers often integrate APIs into their workflows, so making the experience intuitive is key:
- Comprehensive Documentation: Provide clear and concise documentation, ideally generated automatically with tools like Swagger.
- Sample Code: Include simple examples of requests and responses to help developers get started quickly.
4. Optimizing for Performance and Scalability
APIs must handle increasing traffic and growing datasets while maintaining performance. Consider these strategies:
- Fast Response Times: Use efficient data formats like JSON or Protobuf.
- Caching and Load Balancing: Implement caching mechanisms and distribute load to ensure reliability during traffic spikes.
- Independent Scalability: Design APIs so they can scale individually, which is especially important in microservices architectures.
5. Security as a Core Component
API security is non-negotiable. With APIs often being a primary target for attacks, robust protection is essential:
- OAuth 2.0 & JWT: Use proven protocols for authentication and access control.
- TLS Encryption: Protect sensitive data by securing all communication channels with TLS.
- Vulnerability Mitigation: Safeguard against common threats like SQL injection and cross-site scripting (XSS).
6. Managing the API Lifecycle
APIs evolve over time, and a thoughtful lifecycle management approach is crucial:
- Versioning: Use clear versioning schemes (e.g.,
v1
,v2
) to track changes. - Backward Compatibility: Avoid breaking changes to maintain functionality for older integrations.
7. Monitoring and Error Handling
Proactive monitoring and clear error handling are vital for maintaining a stable and reliable API:
- Helpful Error Messages: Return detailed and actionable error codes to assist developers in resolving issues.
- Real-Time Monitoring: Leverage tools like Prometheus or Datadog to keep tabs on performance and detect bottlenecks early.
8. Sustainability and Governance
As API usage grows, maintaining a long-term strategy becomes increasingly important:
- API Governance: Establish clear rules and standards for development and maintenance.
- Regulatory Compliance: Ensure APIs comply with data protection regulations like GDPR.
9. Optimizing for Mobile and Web
APIs must be efficient across platforms and networks. Mobile optimization is particularly critical:
- Minimized Payloads: Transmit only the necessary data to reduce load times.
- Effective Caching: Implement strategies to minimize redundant requests and improve performance.
Practical Example: Building an API with Python
In this section, we’ll walk through building a modern API using Python and Flask. Step by step, we’ll cover setting up the environment, creating the application, and implementing essential API functionalities—all while adhering to modern API design best practices.
Step 1: Setting Up the Environment
Before diving into coding, you’ll need to set up your development environment. First, clone the repository to your local machine:
git clone https://github.com/TechFiora/api-best-practices.git
cd api-best-practices
Once the repository is cloned, create a virtual environment and install the required dependencies using the requirements.txt
file:
# Create a virtual environment (optional, but recommended)
python -m venv venv
# Activate the virtual environment
# On macOS/Linux:
source venv/bin/activate
# On Windows:
venv\Scripts\activate
# Install dependencies
pip install -r requirements.txt
Step 2: Defining the Database Structure
The first step is defining your data models. In models.py, we create the User
model to store user data. This is a critical part of designing an API that is easy to maintain and extend in the future.
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy()
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(50), nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
password = db.Column(db.String(200), nullable=False)
Step 3: Setting Up the Configuration
In config.py we define our application configuration. This includes the database URI, the JWT secret key for authentication, and the cache configuration to help with performance optimization.
class Config:
SQLALCHEMY_DATABASE_URI = "sqlite:///app.db" # SQLite
SQLALCHEMY_TRACK_MODIFICATIONS = False # Disable modification tracking
JWT_SECRET_KEY = "your-secret-key" # Secret key for JWT authentication
CACHE_TYPE = "SimpleCache" # Caching configuration
Step 4: Implementing Authentication
In auth.py, we implement the login functionality, where users authenticate by providing their credentials and receive a JWT token if the credentials are valid.
from flask_jwt_extended import create_access_token
from flask import request, jsonify
from models import User
def login():
username = request.json.get("username", None)
password = request.json.get("password", None)
user = User.query.filter_by(email=username).first()
if user and user.password == password:
access_token = create_access_token(identity=str(user.id))
return jsonify(access_token=access_token)
return jsonify({"message": "Invalid credentials"}), 401
In this code, we follow security best practices by validating credentials securely and issuing JWT tokens for authentication. By using the create_access_token
method from Flask-JWT-Extended, we avoid storing sensitive information like passwords in the client-side application, ensuring that only authenticated users can access protected routes.
Step 5: API Core Logic
Now, in app.py
, we wire everything together: initializing the Flask app, setting up the database and JWT, defining the routes, and adding error handling.
from flask import Flask, jsonify
from flask_jwt_extended import JWTManager, jwt_required
from flask_caching import Cache
from flask_migrate import Migrate
import logging
from models import db, User
from auth import login # Import the login function
# Initialize Flask app
app = Flask(__name__)
app.config.from_object("config.Config")
# Initialize extensions
db.init_app(app)
jwt = JWTManager(app)
migrate = Migrate(app, db)
cache = Cache(app, config={"CACHE_TYPE": "SimpleCache"})
# Setup logging
logging.basicConfig(level=logging.DEBUG)
# Dummy data for development
with app.app_context():
db.create_all()
if User.query.count() == 0:
dummy_user = User(id=1, name="John Doe", email="john.doe@example.com", password="password123")
db.session.add(dummy_user)
db.session.commit()
app.logger.info("Dummy user created")
# Public route to get a user
@app.route("/v1/users/", methods=["GET"])
@cache.cached(timeout=50) # Cache response for performance
def get_user(id):
user = User.query.get(id)
if user is None:
app.logger.error(f"User with id {id} not found")
return jsonify({"message": "User not found"}), 404
return jsonify({"id": user.id, "name": user.name, "email": user.email})
# Login route
@app.route("/login", methods=["POST"])
def login_route():
return login()
# Protected route
@app.route("/protected", methods=["GET"])
@jwt_required() # Requires valid JWT for access
def protected():
return jsonify(message="This is a protected endpoint")
# Error handling
@app.errorhandler(404)
def not_found(error):
app.logger.error(f"404 error: {error}")
return jsonify({"message": "Resource not found"}), 404
@app.errorhandler(500)
def internal_error(error):
app.logger.error(f"500 error: {error}")
return jsonify({"message": "Internal server error"}), 500
# Run the app
if __name__ == "__main__":
app.run(debug=True)
In app.py, several key best practices are applied:
Performance Optimization: By using Flask-Caching, we cache the response for the
/v1/users/<int:id>
route. This ensures that frequently requested data does not hit the database every time, which improves performance and reduces server load.Security: The route
/protected
is secured with JWT authentication. Only users with a valid token can access it. This adds a layer of protection against unauthorized access, ensuring that sensitive data is only accessible to authenticated users.Error Handling and Logging: Proper error handling for
404
and500
errors, along with logging, ensures that issues can be traced and diagnosed easily. This makes the API more reliable and easier to maintain.Scalability: By using Flask-Migrate, we ensure that database migrations are handled smoothly. This helps in scaling the application and managing changes in the database schema over time without downtime.
Once the API is running, you can test its functionality using Postman, a popular tool for API testing. Postman allows you to simulate requests like GET
, POST
, and more, making it easier to validate that your API is working as intended.
For example:
- To test the user retrieval endpoint, send a
GET
request tohttp://127.0.0.1:5000/v1/users/1
. - To test the login functionality, use a
POST
request tohttp://127.0.0.1:5000/login
with the following JSON body:{
"username": "john.doe@example.com",
"password": "password123"
} - To access the protected route, include the JWT token from the login response in the
Authorization
header of aGET
request tohttp://127.0.0.1:5000/protected
.
- To test the user retrieval endpoint, send a
In the next steps, we’ll dive into building app.py
, bringing everything together and adhering to our best practices for a secure, scalable, and intuitive API.